this is part two of our series on building a todo application with htmx and django. click here to view part 1.
In Part 2, we'll create the todo model and implement its basic functionality via unit testing.
Creating the Todo ModelIn models.py, we create the todo model and its basic attributes. We want to associate todo items with a userprofile so that users can only see their own items. A todo item will also have a title and a boolean attribute is_completed. We have many future ideas for the todo model, such as the ability to set tasks to "in progress" in addition to completed or not started, and deadlines, but that's for later. For now, let's keep it simple and get something on the screen as quickly as possible.
Note: In a real-world application, we should probably consider using uuids as primary keys for both the userprofile and todo models, but we'll keep it simple for now.
# core/models.py from django.contrib.auth.models import AbstractUser from django.db import models class UserProfile(AbstractUser): pass class Todo(models.Model): user = models.ForeignKey(UserProfile, on_delete=models.CASCADE) title = models.CharField(max_length=255) is_completed = models.BooleanField(default=False) def __str__(self): return self.title
Let's run migrations for our new model:
❯ uv run python manage.py makemigrations migrations for 'core': core/migrations/0002_todo.py + create model todo ❯ uv run python manage.py migrate operations to perform: apply all migrations: admin, auth, contenttypes, core, sessions running migrations: applying core.0002_todo... okWriting Our First Test
Let's write our first test for the project. We want to ensure that users can only see their own todo items and not those of other users.
To aid in writing our tests, we'll add new development dependencies to our project: model-bakery, which simplifies the process of creating dummy Django model instances, and pytest-django.
❯ uv add model-bakery pytest-django --dev resolved 27 packages in 425ms installed 2 packages in 12ms + model-bakery==1.20.0 + pytest-django==4.9.0
In pyproject.toml, we need to configure pytest by adding a few lines at the end of the file:
# pyproject.toml # new [tool.pytest.ini_options] django_settings_module = "todomx.settings" python_files = ["test_*.py", "*_test.py", "testing/python/*.py"]
Now let's write our first test to ensure that users can only access their own todo items.
# core/tests/test_todo_model.py import pytest @pytest.mark.django_db class TestTodoModel: def test_todo_items_are_associated_to_users(self, make_todo, make_user): [user1, user2] = make_user(_quantity=2) for i in range(3): make_todo(user=user1, title=f"user1 todo {i}") make_todo(user=user2, title="user2 todo") assert {todo.title for todo in user1.todos.all()} == { "user1 todo 0", "user1 todo 1", "user1 todo 2", } assert {todo.title for todo in user2.todos.all()} == {"user2 todo"}
We use a conftest.py file in pytest to hold all the fixtures we plan to use in our tests. The model_bakery library makes creating instances of userprofile and todo simple with minimal boilerplate.
#core/tests/conftest.py import pytest from model_bakery import baker @pytest.fixture def make_user(django_user_model): def _make_user(**kwargs): return baker.make("core.userprofile", **kwargs) return _make_user @pytest.fixture def make_todo(make_user): def _make_todo(user=None, **kwargs): return baker.make("core.todo", user=user or make_user(), **kwargs) return _make_todo
Let's run the tests!
❯ uv run pytest test session starts (platform: darwin, python 3.12.8, pytest 8.3.4, pytest-sugar 1.0.0) django: version: 5.1.4, settings: todomx.settings (from ini) configfile: pyproject.toml plugins: sugar-1.0.0, django-4.9.0 collected 1 item core/tests/test_todo_model.py ✓ 100% ██████████ results (0.25s): 1 passedRegistering the Todos Admin Page
Finally, we can register an admin page for it:
# core/admin.py from django.contrib import admin from django.contrib.auth.admin import UserAdmin from .models import Todo, UserProfile admin.site.register(UserProfile, UserAdmin) admin.site.register(Todo)
We can now add some todos from the admin!
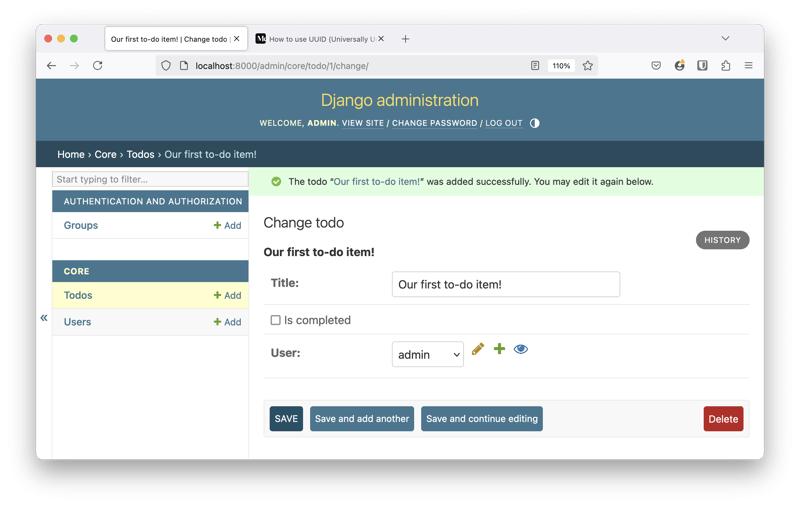
If you want to see the entire code before the end of Part 2, you can check it out on the Part 02 branch on github.
以上就是使用 Django 和 HTMX 创建 To-Do 应用程序 - 使用 TDD 添加 Todo 模型部分的详细内容,更多请关注知识资源分享宝库其它相关文章!
版权声明
本站内容来源于互联网搬运,
仅限用于小范围内传播学习,请在下载后24小时内删除,
如果有侵权内容、不妥之处,请第一时间联系我们删除。敬请谅解!
E-mail:dpw1001@163.com
发表评论